Chapter 13.1: User-defined Data Types
Purpose of user-defined data types:
- To create new data type (from existing data types)
- To allow data types not available in a programming language to be constructed // To extend the flexibility of programming language
Why user-defined data types are necessary:
- No suitable data type is provided by the language used
- The programmer needs to specify a new data type
- … that meets the requirement of the application
State what is meant by user-defined data types:
- Derived from one or more existing data types
- Used to extend the built-in data types
- Creates data types specific to applications
Non-composite Data Types
Define non-composite data types
- A single data type that does not involve a reference to another type / usually built in to a programming language
Give examples of non-composite data type:
- Integer
- Stores as a whole number
- Boolean
- Stores true or false
- Real/double/float/decimal
- Stores decimal numbers
- String
- Stores zero or more characters
- Char
- Stores a single character
- Pointer
- Whole number used to reference a memory location
Enumerated
This is a data type used to store constant values. It is a list of possible values.
⚠: Enumerated 里面不用加引号''
或双引号""
⚠:The values defined in an enumerated data type are ordinal. This mean that enumerated data types have an implied order or values.
Pointer☝
A user-defined non-composite data type referencing a memory location is called a pointer
- Define:
TYPE <pointer> = ^<Typename>
- Declaration:
DECLARE pointerVar : <pointer>
- Reference:
pointerVar <- ^variable
- Dereference:
pointerVar^
The pointer should be defined as follows:
1 | TYPE <pointer> = ^<Typename> |
Combining enumerated data type and pointer data type:
1 | TYPE season = (Spring,Summer,Autumn,Winter) |
Composite Data Types
Define composite data type:
- Data type constructed from other data types
Give examples of composite data types:
- Array
- Indexed collection of items with the same data type
- List
- Indexed collection of items that can have different data type.
- Record
- Collection of related items which may have different data types
- Set
- Stores a finite numbers of different values that have no order // Support mathematical operation
- Class
- Stack
- Queue
- Linked List
- Dictionary
Set🔄
A data type to create sets and apply the mathematical operations defined in set theory
- Contains a collection of data values
- There is no organization of data values within the set
- Duplicate values are now allowed
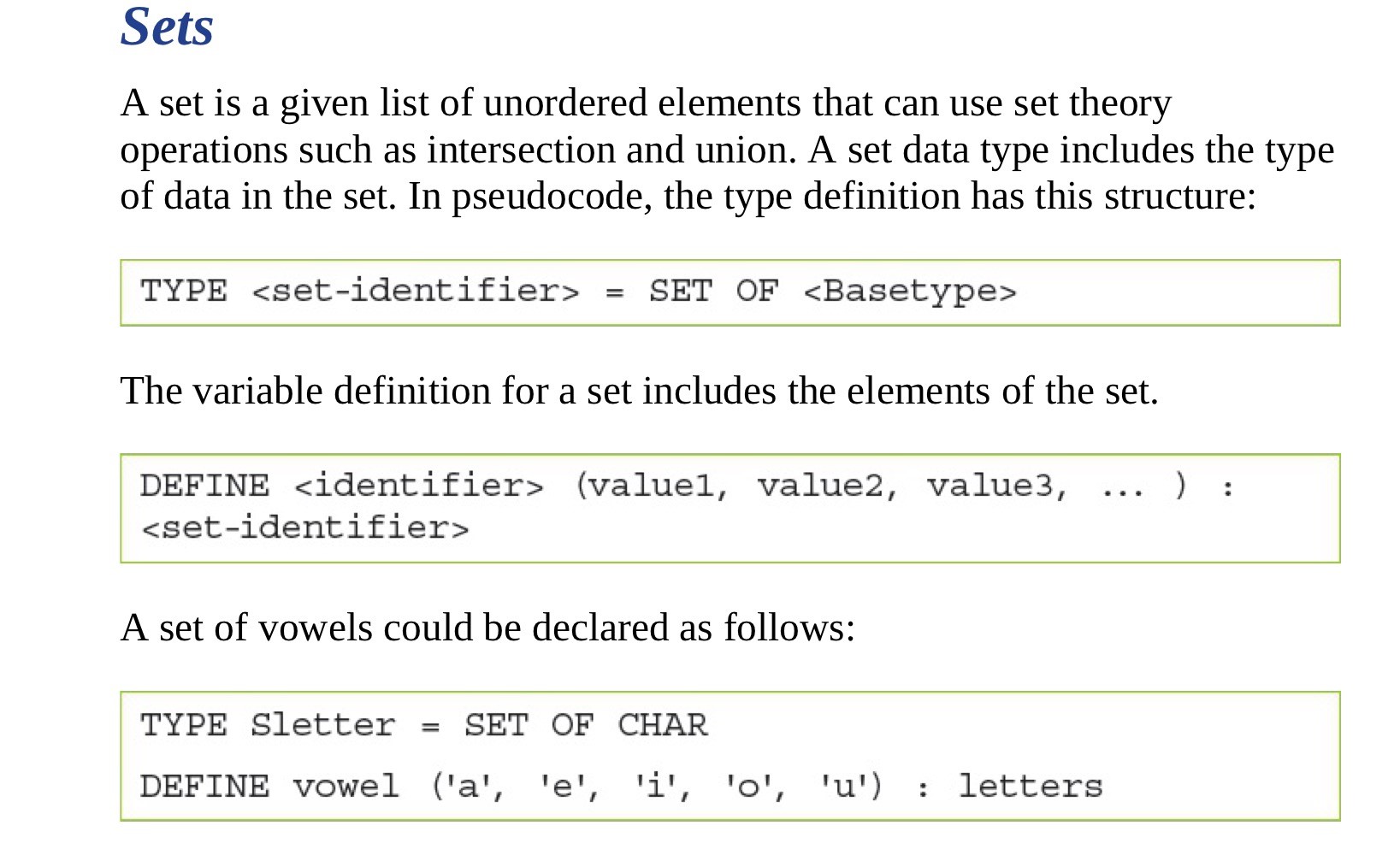
1 | TYPE letter = SET OF CHAR |
Supported operations:
- Check if a value exists in a set
- Adding a new data in set
- Delete a data in set
- Union two sets
Record⏺
1 | TYPE TbookRecord |
Class/object🏛
A template from which all objects are based
Gives the properties and methods for an object
- Attributes: Properties/fields of an object
- Methods: Modules attached to objects to allow it to perform certain actions
Use of User-defined Data Types
Past paper questions
Write pseudocode to create an enumerated type called Parts
to include these parts sold in a computer shop:
Monitor, CPU, SSD, HDD, LaserPrinter, Keyboard, Mouse
1 | TYPE Parts = (Monitor, CPU, SSD, HDD, LaserPrinter, Keyboard, Mouse) |
Write pseudocode to create a pointer type called SelectParts
that will reference the memory location in which the current part name is stored.
1 | TYPE SelectParts = ^Parts |